Course Details-Master Java
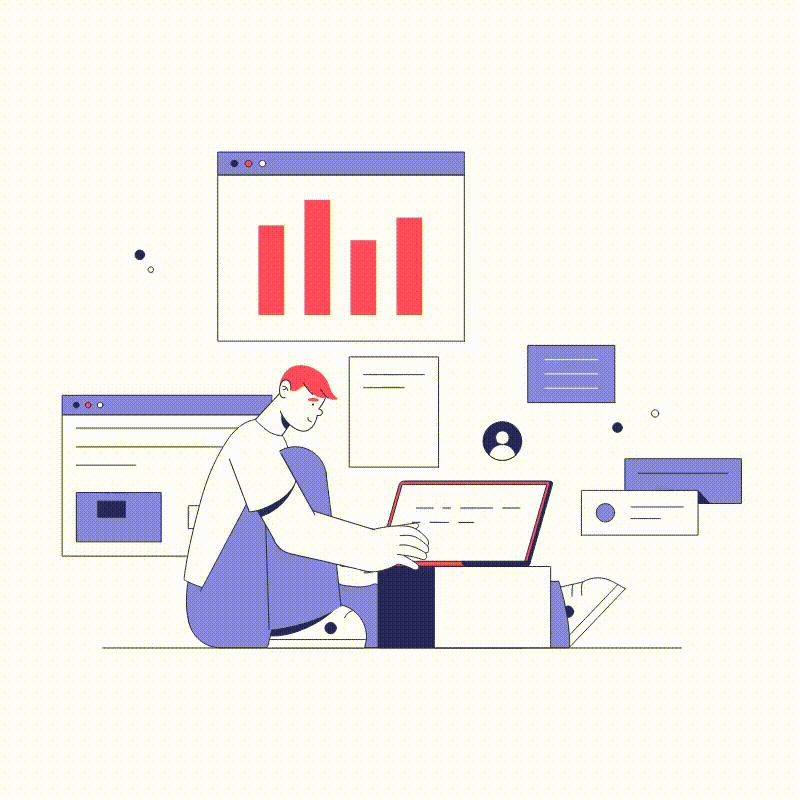
Trainer
Schedule
8.00 pm - 9.00 pm
Available Seats
30
Start Date
21 October 2024
Express interest
Apply Now1. Setting Up Java Development Environment
- Install JDK: Download and install the latest Java Development Kit (JDK) from Oracle or OpenJDK.
- IDE Setup: Set up an Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or VS Code for Java development.
- Build Tools: Use Maven or Gradle for dependency management and build automation.
- Set JAVA_HOME: Set the environment variable to point to the JDK installation directory for proper configuration.
2. Basics of OOP Concepts in Java
- Object-Oriented Principles
- Encapsulation: Binding data (variables) and methods in a single unit (class), providing restricted access.
- Abstraction: Hiding implementation details and exposing only the essential features.
- Inheritance: A mechanism where one class inherits properties and behavior from another class.
- Polymorphism: Ability of objects to take many forms, commonly achieved through method overloading and overriding.
- Classes and Objects: Blueprint and instance of that blueprint, respectively.
- Access Modifiers: Control the visibility of classes, methods, and variables (`public`, `private`, `protected`, default).
- Static vs Non-static Members: Static members belong to the class, while non-static members belong to instances.
- Method Overloading and Overriding: Defining methods with the same name but different parameters or overriding them in subclass.
3. Core Java Concepts
- Data Types, Variables, and Operators: Fundamental building blocks of any Java program, such as `int`, `float`, `boolean`, arithmetic, and logical operators.
- Control Flow Statements: Includes loops (`for`, `while`, `do-while`), conditional statements (`if-else`, `switch`).
- Exception Handling: Mechanisms like `try-catch`, `throw`, `throws` to handle runtime errors and ensure graceful error recovery.
- Collections Framework: A set of interfaces and classes like `List`, `Set`, `Map` to manage groups of objects dynamically.
- Generics: Enables type-safe collections and methods by allowing the use of parameterized types.
4. Streams and File Handling
- Java Streams API: A modern way to process collections of objects in a functional style using methods like `filter()`, `map()`, `reduce()`.
- Intermediate and Terminal Operations: Operations like `filter()`, `sorted()`, `map()` (intermediate) and `forEach()`, `collect()` (terminal) to work on streams.
- Parallel Streams: Processing large data sets in parallel to improve performance.
- File Handling: Reading and writing to files using classes like `FileReader`, `FileWriter`, `BufferedReader`, and Java NIO (`Files`, `Path`).
- Object Serialization: Saving the state of an object into a file and reconstructing it later using `ObjectOutputStream` and `ObjectInputStream`.
5. Real-World Java Application Example: Library Management System
- Entities: Define core entities like `Book`, `Member`, and `Library` to represent the library system.
- CRUD Operations: Implement basic operations for adding, searching, borrowing, and returning books in the library.
- File Handling for Persistence: Store the library’s book and member data using file handling techniques for persistence between sessions.
- Streams for Filtering Data: Use Java Streams to search for books by title, author, or availability status.
- Exception Handling: Handle cases like unavailable books or unregistered members using custom exceptions.