Course Details-SpringBoot
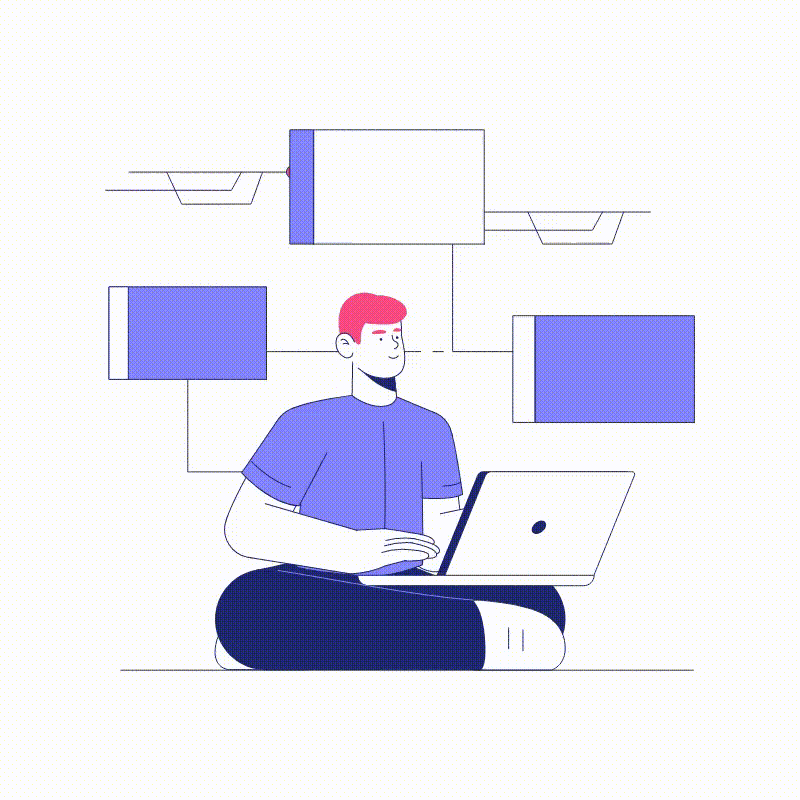
Trainer
Duration
2 Months
Start Date
31st January 2025
Days
3 Days a Week
Time
6.00 pm - 8.00 pm
Certificate
Yes
Pricing
Rs10000 Rs6000
Express interest
Apply Now1. Spring Core Concepts
- Overview of Spring Framework: A comprehensive framework for building Java applications.
- IoC (Inversion of Control) Container: Manages the lifecycle and configuration of application objects.
- Dependency Injection (DI): Injects dependencies into Spring beans to promote loose coupling.
- Spring Bean Lifecycle: Stages of a bean’s lifecycle including instantiation, initialization, and destruction.
- Key Spring Annotations:
- @Component, @Service, @Repository, @Controller
- @Autowired for dependency injection.
- @Configuration, @Bean for configuration.
- @Qualifier to specify which bean to use.
- Spring Boot and Auto-Configuration: Simplifies Spring development by providing auto-configuration and an embedded server.
2. API Development
- RESTful Web Services in Spring Boot: Build REST APIs with Spring Boot annotations.
- Controller Layer: Use @RestController to handle HTTP requests.
- HTTP Methods: Implement `GET`, `POST`, `PUT`, `DELETE` methods.
- Request and Response Handling: Use @RequestBody, @PathVariable, @RequestParam.
- Building RESTful APIs: Create endpoints, handle data using DTOs.
- Validation and Error Handling: Implement validation with @Valid, handle errors with @ControllerAdvice.
- HATEOAS: Implement hypermedia-driven REST API.
3. Database Management
- Spring Data JPA: Simplifies database access with repositories.
- Entity Class and Repositories: Define entities with @Entity and use repository interfaces like CrudRepository.
- Hibernate ORM: Maps Java objects to database tables.
- Database Migrations: Use tools like Flyway or Liquibase for schema versioning and management.
- Connecting to Databases: Configure database connections in application.properties.
- SQL vs NoSQL: Work with relational databases and NoSQL databases like MongoDB.
4. Secure Your Application
- Spring Security Basics: Provides authentication and authorization features.
- Authentication and Authorization: Secure endpoints using user roles and permissions.
- JWT Authentication: Implement JSON Web Token for stateless authentication.
- OAuth2 Integration: Integrate third-party login services using OAuth2.
- CSRF Protection: Protect against Cross-Site Request Forgery attacks.
5. Build and Deploy
- Maven/Gradle for Building: Use Maven or Gradle to compile and package the application.
- Creating Executable JAR or WAR: Package the application as a JAR or WAR file.
- Local and Cloud Deployment: Deploy on local servers or cloud platforms like AWS, Heroku.
- Docker Containerization: Create Docker images for your Spring Boot application.
- CI/CD Pipeline Setup: Automate build, test, and deployment processes using CI/CD tools.